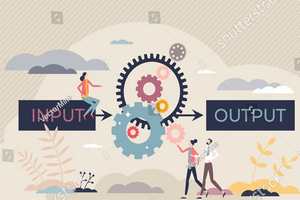
Choose Your Desired Option(s)
# Managing Input and Output in Java
Efficiently managing input and output (I/O) operations is crucial in Java programming, as it allows applications to interact with users, files, and other systems. Java provides a robust set of I/O classes and methods to handle these operations seamlessly. In this article, we’ll explore the essential aspects of managing input and output in Java, including reading from and writing to the console, files, and data streams.
## Console Input and Output
### Reading Input from the Console
Java provides the `Scanner` class to read input from the console. The `Scanner` class is part of the `java.util` package and allows for easy parsing of primitive types and strings.
#### Example of Reading Console Input
“`java
import java.util.Scanner;
public class ConsoleInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter your name: “);
String name = scanner.nextLine();
System.out.println(“Hello, ” + name + “!”);
scanner.close();
}
}
“`
### Writing Output to the Console
For writing output to the console, Java uses the `System.out` object, which is an instance of the `PrintStream` class. Common methods include `print()`, `println()`, and `printf()`.
#### Example of Writing Console Output
“`java
public class ConsoleOutputExample {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
System.out.print(“This is a simple console output.”);
}
}
“`
## File Input and Output
### Reading from a File
Java provides several classes for reading from files, with `FileReader` and `BufferedReader` being the most commonly used. `BufferedReader` is often used for efficient reading of characters, arrays, and lines.
#### Example of Reading a File
“`java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader(“example.txt”))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
“`
### Writing to a File
For writing to files, `FileWriter` and `BufferedWriter` are commonly used. `BufferedWriter` is preferred for writing large amounts of text to a file efficiently.
#### Example of Writing to a File
“`java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class FileWriteExample {
public static void main(String[] args) {
try (BufferedWriter bw = new BufferedWriter(new FileWriter(“example.txt”))) {
bw.write(“Hello, File!”);
bw.newLine();
bw.write(“This is an example of writing to a file.”);
} catch (IOException e) {
e.printStackTrace();
}
}
}
“`
## Data Streams
### Handling Binary Data
Java’s `InputStream` and `OutputStream` classes are used for reading and writing binary data. The `DataInputStream` and `DataOutputStream` classes are specifically designed for handling primitive data types.
#### Example of Writing Binary Data
“`java
import java.io.DataOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class BinaryWriteExample {
public static void main(String[] args) {
try (DataOutputStream dos = new DataOutputStream(new FileOutputStream(“data.bin”))) {
dos.writeInt(123);
dos.writeDouble(3.14);
} catch (IOException e) {
e.printStackTrace();
}
}
}
“`
#### Example of Reading Binary Data
“`java
import java.io.DataInputStream;
import java.io.FileInputStream;
import java.io.IOException;
public class BinaryReadExample {
public static void main(String[] args) {
try (DataInputStream dis = new DataInputStream(new FileInputStream(“data.bin”))) {
int number = dis.readInt();
double decimal = dis.readDouble();
System.out.println(“Number: ” + number);
System.out.println(“Decimal: ” + decimal);
} catch (IOException e) {
e.printStackTrace();
}
}
}
“`
## Conclusion
Managing input and output in Java is essential for building robust applications that can interact with users and external resources. Whether you’re working with console I/O, file I/O, or data streams, Java provides comprehensive tools to handle these tasks efficiently. By mastering these concepts, you can develop applications that effectively manage data and provide a seamless user experience.
Share Now!